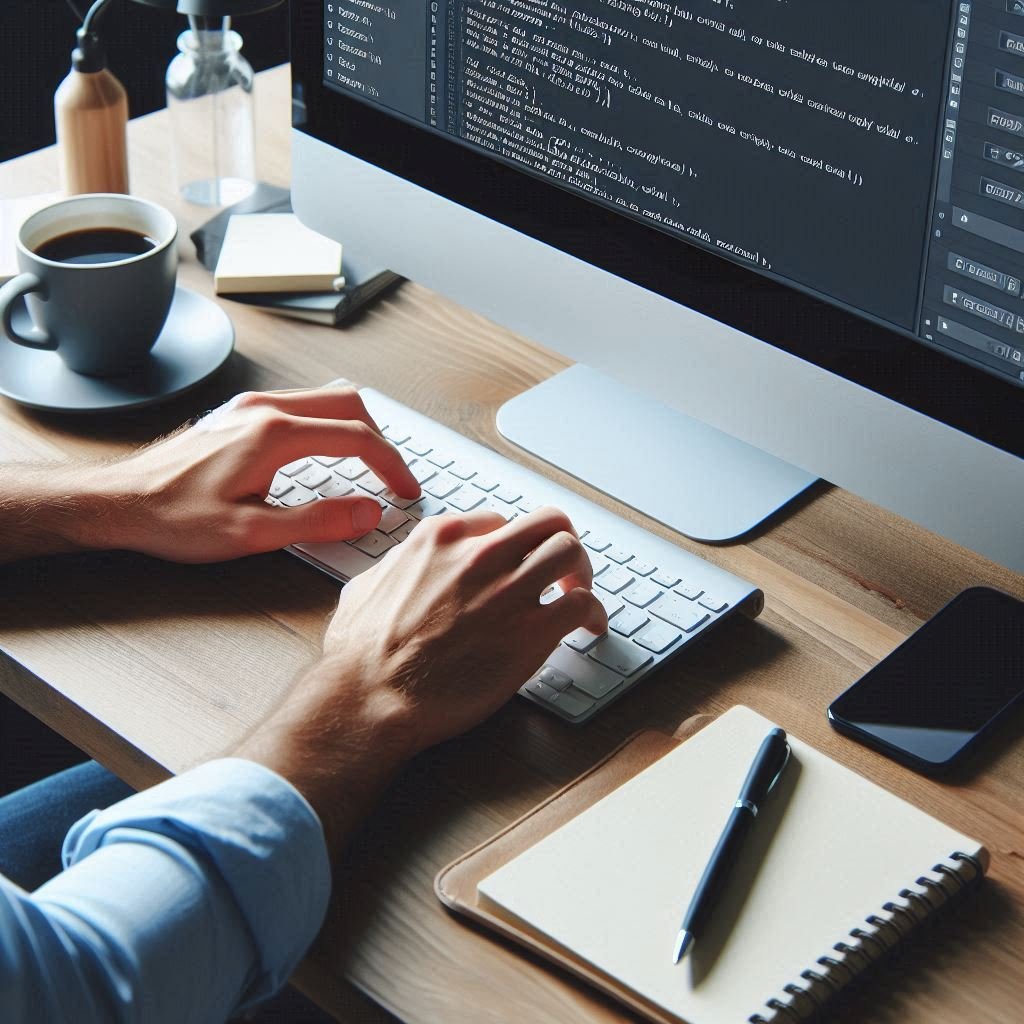
29
Comprehensive Guide to Converting CSV to JSON: A Step-by-Step Approach
Converting CSV (Comma-Separated Values) to JSON (JavaScript Object Notation) is a common requirement in modern data processing, web development, and API integration. CSV is a simple format used to store tabular data that is easily readable by humans and machines. JSON's hierarchical structure and flexibility make it more suitable for complex data representation, especially in web and API contexts.
Introduction:
In data processing and integration, converting CSV (Comma-Separated Values) to JSON (JavaScript Object Notation) has become a prevalent necessity. CSV is a straightforward format for storing tabular data, widely used due to its simplicity and compatibility with various applications.
JSON, on the other hand, offers a more flexible, hierarchical structure, making it ideal for web development, APIs, and data analysis. This comprehensive guide explores the importance of converting CSV to JSON, available tools and methods, and best practices for efficient and accurate conversion.
Can you explain what CSV is?
With commas between each field, data is arranged into rows and columns in the CSV plain text format. The simplicity and usability of this format make it popular for exchanging data between many systems, including databases and spreadsheets.
Structure and Components of a CSV File:
- Header Row: Contains column names.
- Data Rows: Each line represents a row in the table, with fields separated by commas.
Example:
SQL
Copy code
Name, Age, City
Alice, 30, New York
Bob, 25, Los Angeles
Charlie, 35, Chicago
Advantages:
- Simple to read and write.
- Supported by many applications.
- Efficient for storing and sharing tabular data.
Limitations:
- No hierarchical structure.
- Limited support for complex data types.
- Lacks metadata.
What is JSON?
JSON is a simple, lightweight format for exchanging data that is simple for computers to understand and produce as well as for humans to read and write. It represents data as key-value pairs and arrays and supports various data types, including objects, arrays, strings, numbers, booleans, and null.
Structure and Components of a JSON File:
- Objects: Enclosed in curly braces {}, consisting of key-value pairs.
- Arrays: Enclosed in square brackets [], containing ordered values.
Example:
JSON
Copy code
{
"name": "Alice",
"age": 30,
"city": "New York"
}
Advantages:
- Supports hierarchical and nested data.
- Easily integrated with web technologies.
- Language-independent and widely used in APIs.
Limitations:
- It can be more complex than CSV.
- Larger file sizes for the same data.
- Requires more processing power.
Why Convert CSV to JSON?
Converting CSV to JSON is crucial for scenarios where JSON's hierarchical structure and flexibility provide significant advantages over CSV's flat, tabular format. JSON is preferred in web development, data analysis, and API integration due to its ability to represent complex data relationships and compatibility with various programming languages.
Benefits of JSON over CSV:
- Hierarchical Structure: Supports nested data and complex relationships.
- Flexibility: Can store different data types and structures.
- Integration: Widely used in modern web technologies and APIs.
Situations Where JSON is Preferred:
- Web Development: This is for data exchange between the server and the client.
- APIs: APIs are the standard format for API communication.
- Data Analysis: For representing and manipulating complex datasets.
Real-World Applications:
- E-commerce: Converting product data for web applications and APIs.
- Healthcare: Storing and exchanging medical records.
- Finance: Sharing financial data between systems.
Tools for CSV to JSON Conversion
Various tools are available to facilitate CSV to JSON conversion, ranging from online converters to programming libraries.
Online Tools:
- ConvertCSV (convertcsv.com): Quick and easy conversion.
- CSV to JSON Converter (csvjson.com): User-friendly interface for manual conversion.
Software Applications:
- Microsoft Excel: Export CSV and use plugins/scripts for conversion.
- Google Sheets: Export CSV and utilize add-ons/scripts for conversion.
Command-Line Tools:
- CSV to JSON (Node.js): Efficient for batch processing.
- csvkit (Python): Powerful toolkit for CSV manipulation.
Programming Libraries:
- Python: pandas, csv, json.
- JavaScript: PapaParse, json2csv.
- Java: Jackson, Gson.
- Ruby: CSV, JSON.
Manual CSV to JSON Conversion
Manual conversion involves:
- Reading the CSV file.
- Parsing the data.
- Creating JSON objects.
- Writing them to a JSON file.
This process, while straightforward, requires attention to detail to ensure accuracy and efficiency.
Step-by-Step Guide:
- Read the CSV File: Open and read the file line by line.
- Parse the Data: Split each line into fields and organize them.
- Create JSON Objects: Convert the parsed data into JSON format.
- Write to JSON File: Save the JSON objects to a file.
Example: Given CSV data:
SQL
Copy code
Name, Age, City
Alice, 30, New York
Bob, 25, Los Angeles
Charlie, 35, Chicago
Corresponding JSON data:
JSON
Copy code
[
{
"Name": "Alice",
"Age": 30,
"City": "New York"
},
{
"Name": "Bob",
"Age": 25,
"City": "Los Angeles"
},
{
"Name": "Charlie",
"Age": 35,
"City": "Chicago"
}
]
Best Practices:
- Ensure proper handling of special characters and encoding.
- Validate JSON output to avoid errors.
- Optimize JSON for performance by minimizing size.
Automated CSV to JSON Conversion
Automation streamlines the conversion process, making it more efficient and scalable. Scripts and batch processing tools can handle large datasets and repetitive tasks.
Using Scripts:
- Python: Utilize pandas or built-in CSV and JSON libraries.
- JavaScript: Leverage libraries like PapaParse and json2csv.
Batch Processing:
- Automate the conversion of multiple CSV files at once.
- Schedule conversions using cron jobs or task schedulers.
Example in Python:
Python
Copy code
import csv
import json
def csv_to_json(csv_file_path, json_file_path):
data = []
with open(csv_file_path, newline='') as CSV file:
reader = csv.DictReader(CSV file)
for row in reader:
data.append(row)
with open(json_file_path, 'w') as JSON file:
JSON.dump(data, JSON file, indent=4)
csv_to_json('data. csv', 'data.json')
Handling Special Characters and Encodings
Special characters and different encodings can pose challenges during conversion. Correct handling of these is essential to ensure data integrity.
Common Issues:
- Non-ASCII characters.
- Different file encodings (e.g., UTF-8, ISO-8859-1).
Solutions:
- Use libraries that support various encodings.
- Validate and clean data before conversion.
Validating JSON Output
Ensuring the validity of JSON output is crucial for avoiding errors in downstream applications. Various tools and practices can help validate JSON data.
Tools:
- JSONLint (jsonlint.com): Online JSON validator.
- VS Code: Built-in JSON validation.
Common Errors:
- Missing commas or brackets.
- Incorrect data types.
Best Practices:
- Regularly validate JSON during development.
- Use schema validation for complex data structures.
Optimizing JSON for Performance
Optimizing JSON involves minimizing its size and ensuring efficient parsing and generation. This is especially important for large datasets and high-performance applications.
Minimizing Size:
- Remove unnecessary whitespace.
- Use shorter key names.
Best Practices:
- Compress JSON data for transmission.
- Use efficient parsing libraries.
FAQs about CSV to JSON Conversion
- Converting CSV to JSON is often necessary for web development, APIs, and data analysis. JSON's hierarchical structure and flexibility make it more suitable for representing complex data relationships and various data types, facilitating better integration and manipulation in modern applications.
2. What tools can I use to convert CSV to JSON?
There are several tools available for converting Why should I convert CSV to JSON? CSV to JSON, including: - Online tools: Convert CSV to JSON Converter.
- Software applications: Microsoft Excel, Google Sheets.
- Command-line tools: csvtojson (Node.js), csvkit (Python).
- Programming libraries: pandas (Python), PapaParse (JavaScript), Jackson (Java), CSV and JSON libraries (Ruby).
- 3. What are the common challenges when converting CSV to JSON?
Common challenges include handling special characters and different encodings, ensuring the accuracy and validity of the JSON output, and optimizing JSON for performance. Properly managing these issues is important to maintain data integrity and efficiency.
4. How can I automate the CSV to JSON conversion process?
You can automate the conversion process using scripts and batch-processing tools. For example, in Python, you can use the pandas library or built-in csv and JSON libraries to write scripts that read CSV files, convert the data to JSON, and save the output. Automating the process helps manage large datasets and repetitive tasks more efficiently.
5. How can I validate the JSON output to ensure it's correct? Validating JSON output is crucial to avoid errors in downstream applications. Tools like JSONLint provide online validation, while code editors like VS Code offer built-in JSON validation features. To ensure accuracy and correctness, regularly validate your JSON data during development and consider using schema validation for complex data structures.
Contact
Missing something?
Feel free to request missing tools or give some feedback using our contact form.
Contact Us